Vue Js String startswith Method: The Vue.js String startWith() method is used to check whether a string begins with the specified characters or not. This method is useful for checking if a certain substring is present at the start of a string, which can be especially helpful in detecting if a string is a certain type or format . This means that the method returns true if the given string matches exactly with the start of a string; otherwise, it will return false.Here in this tutorial, we will explain how to use the startswith function with vue js, as shown below:
In Vue.js, how do you check if one string begins with another?
Test if a string starts with the alphabet. This can be achieved by using the this.string.startsWith() method in Vue.js.
Vue Js check character startwith
<div id="app">
<button @click="myFunction">click me</button>
<p>Text: {{text}}</p>
<p>Results :{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
text :'Font Awesome icons offer an easy way to integrate stylish graphics into webpages, with a huge library of over 1500 free vector icons and tutorials to help beginners learn more quickly',
results:''
}
},
methods:{
myFunction(){
this.results = this.text.startsWith("Font");
},
}
}).mount('#app')
</script>
Output of above example
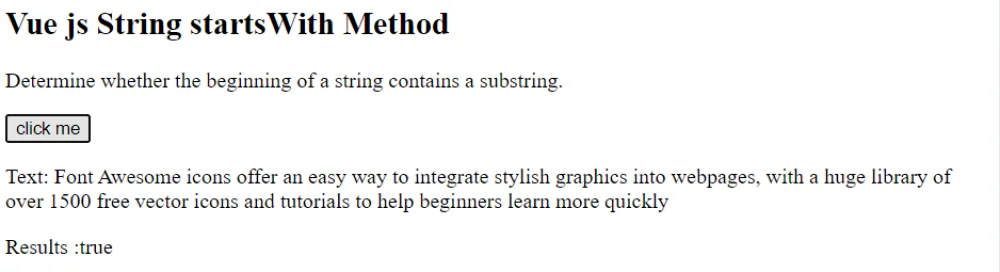
How to check string start with substring start at position 5 in Vue Js ?
Vue.js provides a method to check if a string starts with a substring at the given position This can be achieved by using the this.string.startsWith(subString, 5) method in Vue.js.
Vue Js start at position | Example
<div id="app">
<button @click="myFunction">click me</button>
<p>Text: {{text}}</p>
<p>Results :{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
text :'Font Awesome icons offer an easy way to integrate stylish graphics into webpages, with a huge library of over 1500 free vector icons and tutorials to help beginners learn more quickly',
results:''
}
},
methods:{
myFunction(){
this.results = this.text.startsWith("Awesome",5);
},
}
}).mount('#app')
</script>
Output of above example
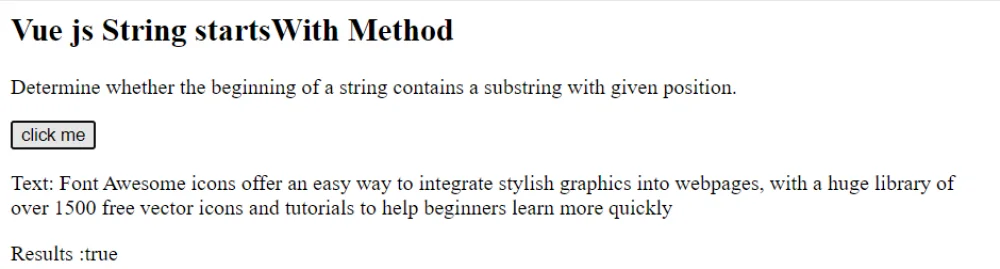